Last active
December 8, 2023 08:55
-
-
Save kyoncy/b662159121ad2ee5523ed4c750193707 to your computer and use it in GitHub Desktop.
Download Blockly.Workspace as SVG or PNG file
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import Blockly from 'blockly'; | |
declare interface CustomNode extends Node { | |
removeAttribute(arg0: string); | |
} | |
const DOMURL = window.URL || window.webkitURL; | |
const getSvgBlob = (workspace: Blockly.WorkspaceSvg) => { | |
const canvas = workspace.svgBlockCanvas_.cloneNode(true) as CustomNode; | |
canvas.removeAttribute("transform"); | |
const css = `<defs><style type="text/css" xmlns="http://www.w3.org/1999/xhtml"><![CDATA[${Blockly.Css.CONTENT.join('')}]]></style></defs>`; | |
const bboxElement = document.getElementsByClassName("blocklyBlockCanvas")[0] as SVGGraphicsElement; | |
const bbox = bboxElement.getBBox(); | |
const content = new XMLSerializer().serializeToString(canvas); | |
const xml = `<svg version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" width="${ | |
bbox.width}" height="${bbox.height}" viewBox=" ${bbox.x} ${bbox.y} ${bbox.width} ${bbox.height}">${ | |
css}">${content}</svg> | |
`; | |
return new Blob([xml], { type: 'image/svg+xml;base64' }); | |
}; | |
const download = (url: string, filename: string) => { | |
const element = document.createElement('a'); | |
element.href = url; | |
element.download = filename; | |
element.click(); | |
DOMURL.revokeObjectURL(element.href); | |
}; | |
export const downloadBlocklySvg = (workspace: Blockly.WorkspaceSvg) => { | |
download(DOMURL.createObjectURL(getSvgBlob(workspace)), 'blocks.svg'); | |
}; | |
export const downloadBlocklyPng = (workspace: Blockly.WorkspaceSvg) => { | |
const img = new Image(); | |
img.onload = () => { | |
const canvas = document.createElement('canvas'); | |
canvas.width = 800; | |
canvas.height = 600; | |
canvas.getContext("2d").drawImage(img, 0, 0); | |
download(canvas.toDataURL("image/png"), 'blocks.png'); | |
}; | |
img.src = DOMURL.createObjectURL(getSvgBlob(workspace)); | |
}; |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
This almost works for me, but some gradient or path in the resulting SVG is off. These are the blocks on the SVG workspace:
This is how the SVG is rendered:
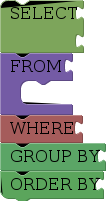
Do you have any idea why that happens? The resulting SVG code reads as follows:
And is there any chance you could explicitly attach a permissive License to this? My own code is AGPL 3 and I would love to use your snippet.